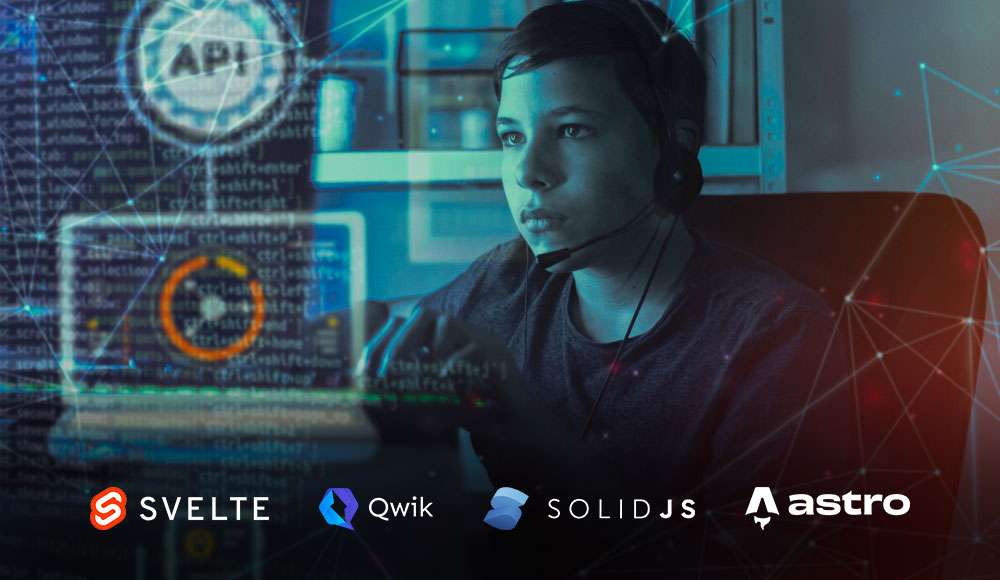
In the ever-evolving world of web development, frameworks are constantly being created to make the development process faster, more efficient, and more enjoyable. While popular frameworks like React, Angular, and Vue.js continue to dominate the scene, newer frameworks are beginning to gain traction by offering innovative solutions for performance, simplicity, and scalability.
In this blog, we’ll explore four modern web development frameworks that have been creating buzz in the developer community: Svelte, Qwik, Astro, and SolidJS. These frameworks offer different approaches to building web applications, from reactive paradigms to hybrid server-side rendering models, and are worth understanding for any developer looking to stay ahead of the curve.
Hire a Developer
Svelte: The Compiler-Based Framework
What is Svelte?
Svelte is a revolutionary framework that takes a different approach from traditional JavaScript frameworks like React or Vue. Instead of using a virtual DOM, Svelte compiles your app into efficient, imperative code that directly manipulates the DOM. This compilation step happens at build time, which results in a much faster runtime and less JavaScript to send over the network.
Key Features
- No Virtual DOM: Unlike React or Vue, Svelte does not use a virtual DOM to track changes. Instead, it compiles components into highly optimized JavaScript code that updates the DOM directly.
- Small Bundle Size: Svelte’s compiler ensures that only the necessary JavaScript is included in the final output, leading to smaller bundle sizes and faster loading times.
- Reactive Programming: Svelte has a built-in reactive programming model that simplifies state management. You can declare reactive variables that automatically update the UI when the state changes.
- Ease of Use: Svelte’s syntax is simple and intuitive. Developers don’t need to deal with hooks, context, or component lifecycle methods like in React. The reactive paradigm feels more natural and direct.
Why Use Svelte?
- Performance: By compiling components to efficient JavaScript code, Svelte apps tend to be faster and more lightweight compared to traditional JavaScript frameworks.
- Simplicity: Its declarative, reactive syntax makes it easy for developers to understand and use. Svelte doesn’t require much boilerplate code or complex configuration.
- Smaller Bundle Size: Since Svelte doesn’t rely on a virtual DOM, apps are typically smaller and have a smaller footprint. This means faster initial loading times.
Use Case
Svelte is ideal for building high-performance, single-page applications (SPAs), dashboards, and any application where performance and bundle size are critical factors.
Example of Svelte Code
<script>
let count = 0;
</script>
<button on:click={() => count += 1}>
Count: {count}
</button>
In this example, the variable count is automatically reactive, and the DOM is updated whenever its value changes, without any need for manual DOM updates or reconciliation.
Popular Apps Built with Svelte
- The New York Times: Some of their interactive features are powered by Svelte.
- Rakuten: A large e-commerce platform uses Svelte for its lightweight and performant nature.
Qwik: The Instant Loading Framework
What is Qwik?
Qwik is a new and innovative framework designed to prioritize instant loading and server-side rendering (SSR). It focuses on delivering ultra-fast performance by optimizing how components are loaded and executed. Qwik allows pages to load instantaneously by breaking down the application into smaller chunks that load only when needed, enabling the concept of resumability — the ability to resume a web app from the server state without re-executing code.
Key Features
- Resumability: Qwik can resume the execution of a web app directly from the server’s state, allowing it to avoid reloading or re-executing JavaScript. This drastically improves the time-to-interactivity (TTI).
- Fine-Grained Lazy Loading: Qwik automatically splits the app into extremely fine-grained chunks, which can be lazy-loaded based on user interaction.
- Zero JavaScript Overhead: Initially, Qwik can send zero JavaScript to the client. The app remains functional, and JavaScript is only fetched as needed.
- Automatic Server-Side Rendering: Qwik provides built-in support for SSR, making it great for SEO, as the server pre-renders HTML and sends it to the browser for fast initial page loads.
Why Use Qwik?
- Super Fast Loading: Qwik’s ability to deliver instant loading with fine-grained lazy loading sets it apart from other frameworks.
- Reduced JavaScript Execution: Since Qwik focuses on execution on the server, the client-side JavaScript is minimal, leading to faster time-to-interactive (TTI).
- Great for SEO: By providing built-in server-side rendering, Qwik ensures that search engines can easily index your app.
Use Case
Qwik is especially suitable for building high-performance, SEO-friendly, content-heavy websites or apps where page speed and initial loading times are paramount.
Example of Qwik Code
import { component$ } from '@builder.io/qwik';
export const Counter = component$(() => {
const [count, setCount] = useState(0);
return (
<button onClick$={() => setCount(count + 1)}>
Count: {count}
</button>
);
});
In this example, component$ defines a Qwik component, and the onClick$ handler is optimized to only load the necessary part of the code when the event happens.
Popular Apps Built with Qwik
- Builder.io: The creators of Qwik have used it to build their own platform, ensuring the framework’s capabilities are battle-tested.
Astro: The Static Site Generator with a Modern Twist
What is Astro?
Astro is a new front-end framework designed for building static websites with ease, focusing on performance by allowing developers to use components from various frameworks (like React, Vue, Svelte, and SolidJS) in a single project. Astro’s main feature is partial hydration, meaning only the parts of the page that need to be interactive get JavaScript, and the rest of the page is rendered as static HTML.
Key Features
- Partial Hydration: Astro only hydrates interactive components with JavaScript when needed. Non-interactive content is rendered as static HTML.
- Multi-Framework Support: You can mix and match components from various frameworks (React, Vue, Svelte, SolidJS, etc.) in a single Astro project.
- Zero JavaScript by Default: Astro generates static HTML by default, and JavaScript is only added when necessary (for interactive components).
- Built-in Markdown Support: Ideal for building content-heavy sites like blogs, documentation sites, and marketing pages.
Why Use Astro?
- Performance First: Since Astro defaults to static site generation and only sends JavaScript when absolutely necessary, it offers exceptional performance out of the box.
- Framework Flexibility: Astro allows developers to use the best parts of multiple frameworks in one project, which is useful if you’re already familiar with React or Vue but want to leverage Astro’s performance benefits.
- Perfect for Content Sites: Astro is an excellent choice for building blogs, documentation, portfolios, and other content-heavy websites where SEO and performance matter.
Use Case
Astro is ideal for building static websites, blogs, marketing pages, documentation sites, and even e-commerce websites where SEO and load speed are crucial.
Example of Astro Code
// This is an Astro component using React
import { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return <button onClick={() => setCount(count + 1)}>{count}</button>;
};
---
<Counter client:load />
In this example, the React Counter component is rendered client-side only when needed.
Popular Apps Built with Astro
- Smashing Magazine: A popular web design publication that uses Astro to optimize their page load times.
- Apple Developer: Apple’s Developer documentation site is powered by Astro for speed and scalability.
SolidJS: The Fast and Reactive UI Library
What is SolidJS?
SolidJS is a declarative UI library that is similar to React but focused on performance and reactivity. It takes advantage of fine-grained reactivity (similar to Svelte) and renders updates directly to the DOM without the need for a virtual DOM. SolidJS is often praised for its speed and small bundle size, making it one of the fastest UI libraries available today.
Key Features
- Fine-Grained Reactivity: SolidJS uses a fine-grained reactivity system that allows it to update only the parts of the UI that have changed, making it highly performant.
- No Virtual DOM: SolidJS directly updates the DOM, which reduces the overhead introduced by a virtual DOM.
- React-like API: SolidJS shares much of its API with React, making it easy for React developers to transition to SolidJS.
- Minimalist: Unlike other frameworks, SolidJS doesn’t come with a lot of built-in abstractions, which leads to a smaller footprint and a more flexible approach to UI development.
Why Use SolidJS?
- Extreme Performance: SolidJS’s fine-grained reactivity and direct DOM manipulation make it extremely fast, especially for high-interactivity applications.
- Small Bundle Size: SolidJS is designed to be as lightweight as possible, making it an ideal choice for performance-sensitive applications.
- React Compatibility: For developers familiar with React, SolidJS offers a similar development experience with improved performance.
Use Case
SolidJS is a great choice for building highly interactive user interfaces where performance is critical.
Example of SolidJS Code
import { createSignal } from 'solid-js';
function Counter() {
const [count, setCount] = createSignal(0);
return (
<button onClick={() => setCount(count() + 1)}>
Count: {count()}
</button>
);
}
In this example, createSignal creates a reactive state variable, and SolidJS updates the DOM whenever the state changes.
Popular Apps Built with SolidJS
- SolidJS itself: The SolidJS website and documentation are built using SolidJS, showcasing its capabilities in action.
Conclusion: Choosing the Right Framework
Each of the frameworks covered in this blog – Svelte, Qwik, Astro, and SolidJS—offers unique advantages and is suited to different use cases:
- Svelte: Great for high-performance, reactive applications with a simple and intuitive syntax.
- Qwik: Ideal for ultra-fast, instant-loading web applications with a focus on fine-grained lazy loading and server-side rendering.
- Astro: Perfect for static websites and content-heavy applications that need fast loading and SEO optimization with minimal JavaScript.
- SolidJS: Best for performance-critical applications that need fine-grained reactivity and fast updates without a virtual DOM.
These modern frameworks represent a new wave of tools designed to solve common web development challenges like performance, bundle size, and interactivity. As they continue to evolve, we can expect to see even more exciting innovations in the world of front-end development.