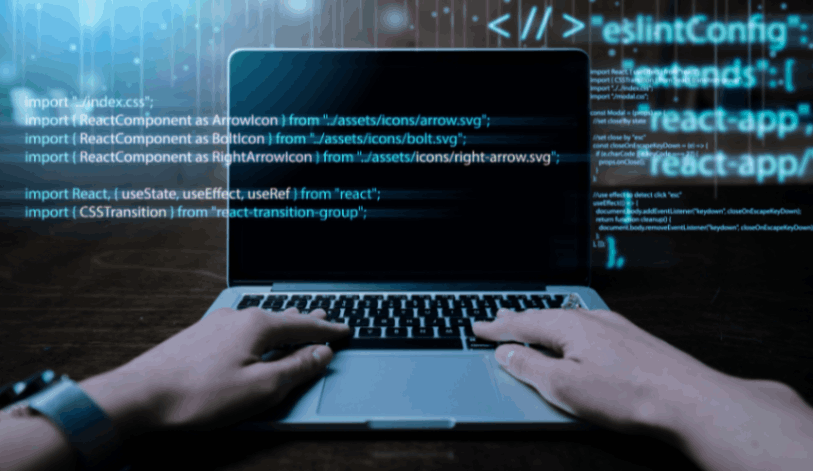
Laravel is one of the most popular PHP frameworks, and one of its standout features is the Eloquent ORM (Object-Relational Mapping). Eloquent makes working with databases extremely simple and enjoyable by providing an expressive, fluent interface for interacting with the database. In this blog post, we’ll explore the basics of Eloquent ORM and delve into some advanced features that can enhance your Laravel applications.
Hire a Laravel Developer
What is Eloquent ORM?
Eloquent ORM is Laravel’s built-in ORM that provides a straightforward and easy-to-use ActiveRecord implementation for working with databases. Each database table has a corresponding “Model” that is used to interact with that table. Models allow you to query for data in your tables, as well as insert new records into the table.
Getting Started with Eloquent ORM
To start using Eloquent, you need to set up a Laravel project and configure your database connection.
Step 1: Setting Up a Laravel Project
First, you need to create a new Laravel project. You can do this using Composer:
composer create-project --prefer-dist laravel/laravel blog
Step 2: Configuring the Database
Open the .env file in your project root and update the database configuration to match your database settings:
env
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database_name
DB_USERNAME=your_database_user
DB_PASSWORD=your_database_password
Step 3: Creating a Model and Migration
Let’s create a simple Post model along with a migration file. Run the following Artisan command:
php artisan make:model Post -m
This command will create a Post model in the app/Models directory and a migration file in the database/migrations directory.
Step 4: Defining the Schema
Open the migration file and define the schema for the posts table:
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreatePostsTable extends Migration
{
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('posts');
}
}
Run the migration to create the posts table in your database:
php artisan migrate
Basic Usage of Eloquent ORM
Now that we have set up our Post model and database table, let’s look at how to perform basic CRUD (Create, Read, Update, Delete) operations using Eloquent.
Creating Records
To create a new record in the posts table, you can use the create method:
use App\Models\Post;
$post = Post::create([
'title' => 'My First Post',
'content' => 'This is the content of my first post.'
]);
Ensure that the fillable property is set in your model to allow mass assignment:
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
use HasFactory;
protected $fillable = ['title', 'content'];
}
Reading Records
To retrieve records from the posts table, you can use methods such as all, find, and where:
// Get all posts
$posts = Post::all();
// Get a single post by ID
$post = Post::find(1);
// Get posts with a specific condition
$posts = Post::where('title', 'My First Post')->get();
Updating Records
To update a record, first retrieve it and then call the update method:
$post = Post::find(1);
$post->update([
'title' => 'Updated Title',
'content' => 'Updated content.'
]);
Deleting Records
To delete a record, retrieve it and call the delete method:
$post = Post::find(1);
$post->delete();
Advanced Features of Eloquent ORM
Eloquent ORM offers several advanced features that can make working with your database even more powerful and expressive.
Eloquent Relationships
Eloquent makes managing and interacting with relationships between your tables simple and intuitive. Here are the most common types of relationships:
- One to One
- One to Many
- Many to Many
- Has Many Through
- Polymorphic Relationships
One to One
A one-to-one relationship is used to define a single related model. For example, if each user has one profile, you can define this relationship in the User and Profile models.
// User Model
public function profile()
{
return $this->hasOne(Profile::class);
}
// Profile Model
public function user()
{
return $this->belongsTo(User::class);
}
One to Many
A one-to-many relationship is used to define multiple related models. For example, a post can have many comments.
// Post Model
public function comments()
{
return $this->hasMany(Comment::class);
}
// Comment Model
public function post()
{
return $this->belongsTo(Post::class);
}
Many to Many
A many-to-many relationship is more complex and involves an intermediate table. For example, a user can have many roles and a role can belong to many users.
// User Model
public function roles()
{
return $this->belongsToMany(Role::class);
}
// Role Model
public function users()
{
return $this->belongsToMany(User::class);
}
Eager Loading
Eager loading is used to minimize the number of queries executed and to load related models along with the main model. This can be achieved using the with method:
$posts = Post::with('comments')->get();
Accessors and Mutators
Accessors and mutators allow you to format Eloquent attributes when retrieving or setting their values.
Accessors
An accessor is used to format the value when retrieving it:
public function getTitleAttribute($value)
{
return ucfirst($value);
}
Mutators
A mutator is used to format the value before saving it to the database:
public function setTitleAttribute($value)
{
$this->attributes['title'] = strtolower($value);
}
Query Scopes
Query scopes allow you to define common query constraints that you can reuse throughout your application.
// Local Scope
public function scopePopular($query)
{
return $query->where('views', '>', 100);
}
// Usage
$popularPosts = Post::popular()->get();
Conclusion
Eloquent ORM is a powerful tool that simplifies database interactions in Laravel applications. Its expressive syntax and robust feature set allow you to handle complex database operations with ease. Whether you’re just getting started with Laravel or looking to deepen your understanding of Eloquent, mastering its basics and advanced features will undoubtedly enhance your development workflow.
By leveraging Eloquent’s relationships, eager loading, accessors, mutators, and query scopes, you can build efficient, maintainable, and scalable applications.