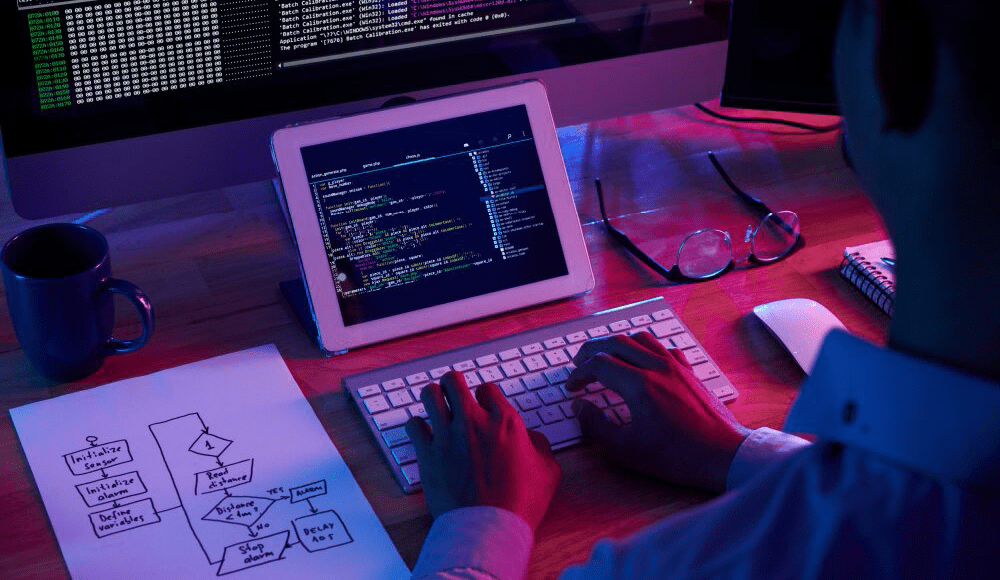
Laravel, a powerful PHP framework, is known for its elegant syntax and robust features. At its core lies the Model-View-Controller (MVC) architecture, which helps in organizing and managing code efficiently. This blog delves into the details of Laravel’s MVC architecture, explaining each component and how they interact to create dynamic web applications.
Hire a Laravel Developer
-
Introduction to MVC Architecture
- Model: Manages data and business logic.
- View: Handles the presentation layer, displaying data to the user.
- Controller: Acts as an intermediary between Model and View, processing user input and updating both Model and View accordingly.
-
The Role of the Model in Laravel
- Interacting with the database.
- Fetching, inserting, updating, and deleting records.
- Implementing business logic.
-
The Role of the View in Laravel
- Displaying data to the user.
- Rendering HTML, CSS, and JavaScript.
-
The Role of the Controller in Laravel
- User input.
- Application logic.
- Requests and responses.
-
Interactions Between MVC Components
- User Requests a URL: The user requests a URL which is routed to a specific Controller action.
- Controller Processes Request: The Controller processes the request, interacting with the Model to retrieve or manipulate data.
- Controller Passes Data to View: The Controller passes the data to a View.
- View Renders Data: The View renders the data into HTML and sends it back to the user’s browser.
- Route: A user visits /posts, which is routed to the index method of PostController.
- Controller: PostController@index retrieves all posts from the Post Model.
- Model: Post::all() fetches all posts from the database.
- View: The data is passed to posts.index View, which renders the posts.
-
Advantages of Using MVC in Laravel
-
Practical Example: Building a Simple Blog
MVC stands for Model-View-Controller, a design pattern used to separate the concerns of an application into three interconnected components:
This separation of concerns promotes organized, maintainable, and scalable code.
What is the Model?
The Model represents the data layer of the application. It is responsible for:
Laravel’s Eloquent ORM
Laravel uses Eloquent ORM (Object-Relational Mapping) to interact with databases. Eloquent provides a simple and intuitive ActiveRecord implementation to work with your database.
Example
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
protected $fillable = ['title', 'content'];
// Define relationships, scopes, and other business logic
}
In the example above, Post is a Model representing a blog post. It extends the Model class provided by Eloquent, and the $fillable property specifies which attributes can be mass-assigned.
What is the View?
The View is the presentation layer. It is responsible for:
Blade Templating Engine
Laravel uses Blade, a powerful templating engine, to create dynamic and reusable views.
Example
Blade file
<!-- resources/views/posts/index.blade.php -->
@extends('layouts.app')
@section('content')
<h1>Blog Posts</h1>
@foreach ($posts as $post)
<div class="post">
<h2>{{ $post->title }}</h2>
<p>{{ $post->content }}</p>
</div>
@endforeach
@endsection
In the example above, Blade’s syntax is used to loop through a collection of posts and display their title and content.
What is the Controller?
The Controller acts as the intermediary between the Model and the View. It handles:
Example
namespace App\Http\Controllers;
use App\Models\Post;
use Illuminate\Http\Request;
class PostController extends Controller
{
public function index()
{
$posts = Post::all();
return view('posts.index', compact('posts'));
}
public function store(Request $request)
{
$validated = $request->validate([
'title' => 'required|max:255',
'content' => 'required',
]);
Post::create($validated);
return redirect()->route('posts.index');
}
}
In the example above, the PostController has methods to handle displaying all posts (index) and storing a new post (store).
Workflow
Example Workflow
Separation of Concerns
MVC separates the application into distinct components, making it easier to manage and maintain.
Scalability
As the application grows, the separation of concerns makes it easier to scale by adding more Models, Views, and Controllers.
Reusability
Code in Models and Controllers can be reused across different parts of the application, while Views can be made reusable using Blade templates and components.
Testability
Having distinct components makes it easier to write unit tests and functional tests, ensuring the application behaves as expected.
Step 1: Setting Up Routes
// routes/web.php
use App\Http\Controllers\PostController;
Route::get('/posts', [PostController::class, 'index'])->name('posts.index');
Route::post('/posts', [PostController::class, 'store'])->name('posts.store');
Step 2: Creating the Model
// app/Models/Post.php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
protected $fillable = ['title', 'content'];
}
Step 3: Creating the Controller
// app/Http/Controllers/PostController.php
namespace App\Http\Controllers;
use App\Models\Post;
use Illuminate\Http\Request;
class PostController extends Controller
{
public function index()
{
$posts = Post::all();
return view('posts.index', compact('posts'));
}
public function store(Request $request)
{
$validated = $request->validate([
'title' => 'required|max:255',
'content' => 'required',
]);
Post::create($validated);
return redirect()->route('posts.index');
}
}
Step 4: Creating the View
<!-- resources/views/posts/index.blade.php -->
@extends('layouts.app')
@section('content')
<h1>Blog Posts</h1>
<form action="{{ route('posts.store') }}" method="POST">
@csrf
<div>
<label for="title">Title:</label>
<input type="text" id="title" name="title" required>
</div>
<div>
<label for="content">Content:</label>
<textarea id="content" name="content" required></textarea>
</div>
<button type="submit">Create Post</button>
</form>
@foreach ($posts as $post)
<div class="post">
<h2>{{ $post->title }}</h2>
<p>{{ $post->content }}</p>
</div>
@endforeach
@endsection
Conclusion
Understanding Laravel’s MVC architecture is fundamental to developing clean, efficient, and maintainable web applications. By separating the application into Models, Views, and Controllers, developers can manage complexity and facilitate collaboration. Laravel’s robust tools, like Eloquent and Blade, further streamline the development process, making it a powerful framework for modern web development.